California’s CCPA and CCRA require opt-in to share or sell personal data, so make sure you offer that setting alongside your existing cookie options. If you happen to use OneTrust, here’s how that looks:
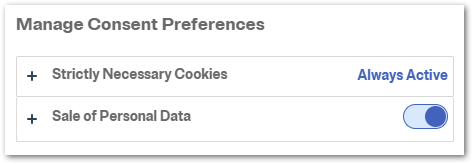
That’ll store the setting in OneTrust’s internal database and in their local cookie, but how do you push it to Marketo when the visitor becomes known?
It’s undocumented but turns out to be really simple.
First pass: Decode the cookie as if it’s a query string
The OneTrust cookie is called OptanonConsent
. It has a predictable, standard encoding: exactly like a URL query string or application/x-www-form-urlencoded
request.
Here’s an example OptanonConsent
cookie value (yes, the whole thing is the value of a single cookie):
isGpcEnabled=0&datestamp=Sat+Jan+13+2024+20%3A24%3A04+GMT-0500+(Eastern+Standard+Time)&version=202308.1.0&browserGpcFlag=0&isIABGlobal=false&hosts=&consentId=5c7582be-2173-4c6d-b027-5471792054b3&interactionCount=1&landingPath=NotLandingPage&groups=1%3A1%2C3%3A1%2CSPD_BG%3A1%2C2%3A1%2C4%3A1
Say you have that cookie value in a variable optanonCookie
.
URL-decode just as you would a query string.
let optanonStoredData = new URLSearchParams(optanonCookie);
Second pass: Parse the query param groups
optanonStoredData
is a URLSearchParams object (my favorite quirky variant of a JS Map). It has a key named groups
whose value is a comma-delimited list of colon-delimited key:value pairs:
1:1,3:1,SPD_BG:1,2:1,4:1
Parse that value into a usable Map like this:
let optanonGroups = new Map(optanonStoredData.get("groups").split(",").map((entry) => entry.split(":")));
Third pass: Get the OneTrust settings from the parsed groups
optanonGroups
is a Map. Its keys are the OneTrust group IDs (which can be all-numeric strings or alphanumeric strings). Each value is either string "0"
for disabled or string "1"
for enabled.
You can get group IDs from the popup HTML. See where group "1"
is the Strictly Necessary Cookies setting, while group "SPD_BG"
corresponds to Sale of Personal Data?
So get the Sale of Personal Data group like so:
let optanonSPDGroup = optanonGroups.get("SPD_BG"); // returns "1" for the cookie above
Writing the settings to Marketo form fields
This Forms 2.0 JS updates the custom Marketo fields OneTrust Sell Personal Data and OneTrust Timestamp:
MktoForms2.onSubmit(function(submittingForm){
let cookieReader = new URLSearchParams( document.cookie.split("; ").map( kv => [kv.split("=")].flatMap( ([k, ...v]) => [k, v.join("=")] ) ) );
let optanonCookie = cookieReader.get("OptanonConsent");
let optanonSections = new URLSearchParams(optanonCookie);
let optanonGroupsSection = optanonSections.get("groups");
let optanonDatestamp = optanonSections.get("datestamp");
let optanonGroups = new Map(optanonGroupsSection.split(",").map( entry => entry.split(":")) );
submittingForm.addHiddenFields({
OneTrustSellPersonalData : optanonGroups.get("SPD_BG"),
OneTrustTimestamp: new Date(optanonDatestamp).toISOString()
});
});
You can extract any OneTrust group from optanonGroups
the same way.
P.S. I’ll describe that cookieReader
logic more in an upcoming post — your existing cookie parsing code most likely has a bug, but this code doesn’t! 😛