I’m no fan of the typical way to send anniversary/birthday emails, which relies on a long-running trigger campaign and Advanced Wait Properties. Having hundreds of thousands of leads waiting in different trigger campaigns for 364 days, to use a technical term, creeps me out.
Far more reasonable, I think, is having a daily batch campaign that checks for those people whose anniversary is today. For everyone that qualifies, you set their Next Anniversary Date (a custom Date field) to {{Lead.Next Anniversary Date}} + 1 year using Change Data Value.
The catch, though, is you need to kick off Next Anniversary Date from the right date. From that point forward, everything works automatically. But if you don’t have an external system (or CRM) setting Next Anniversary Date for you, you have to compute it.
Let’s say someone is created on 6/1/2022 with the standard Date of Birth field equal to 12/12/1990. Your custom field Next Birthday should be set to 12/12/2022 so the batch can take over.
Here’s where FlowBoost comes in.
This FlowBoost payload will return both nextBirthday
(DateTime) and nextBirthDateOnly
(Date) from the Date of Birth:
// get today at midnight UTC
let today = new Date();
["setUTCHours","setUTCMinutes","setUTCSeconds","setUTCMilliseconds"].forEach( unitSetter => today[unitSetter](0) );
// convert original DOB to Date
let dateOfBirth = new Date({{Lead.Date of Birth}});
// clone the DOB to a new Date for clarity
var nextBirthday = new Date(dateOfBirth);
// first set to the same day in the current year
nextBirthday.setYear(today.getFullYear());
// only if nextBirthday is still in the past, set one more year forward
if( nextBirthday < today ) {
nextBirthday.setYear(nextBirthday.getFullYear() + 1);
}
// return the date-only version as well (yyyy-MM-dd)
var nextBirthdayDateOnly = nextBirthday.toLocaleDateString("se");
So:
- create a Date object from the Date of Birth
- clone that object to a new Date for manipulation
- set the new Date’s year to this year (keeping month & day the same)
- do a final check to see if you need to tack on another year based on the current month & day
This is just one way to do this in JS, but alternative implementations are welcome!
Cloning Date objects is fun
A notable feature of the Date()
constructor: if you pass it an existing Date object (as opposed to a Date-like String or Number) it makes a deep clone to a new Date. You can then treat the “base” Date as if it’s immutable and only make changes in the clone.
As MDN explains:
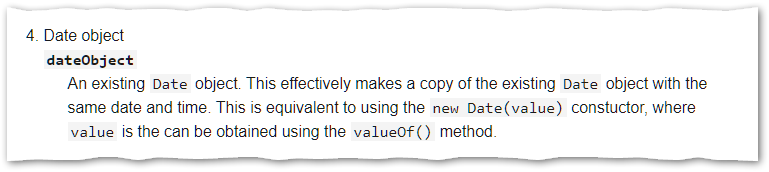
Or if you like explanations that are almost impossible to read, read the ECMAScript spec for Date (the relevant part is “...and value has a [[DateValue]] internal slot...”).